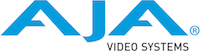 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
8 #ifndef NTV2FRAMEGRABBER_H
9 #define NTV2FRAMEGRABBER_H
11 #include <QBasicTimer>
13 #if (QT_VERSION >= QT_VERSION_CHECK(5, 0, 0))
19 #if (QT_VERSION >= QT_VERSION_CHECK(6, 0, 0))
20 #include <QtMultimedia>
22 #include <QAudioOutput>
30 #if defined (INCLUDE_AJACC)
33 #endif // INCLUDE_AJACC
35 #define QTPREVIEW_WIDGET_X (960)
36 #define QTPREVIEW_WIDGET_Y (540)
70 inline void SetWithAudio (
const bool inWithAudio) {mbWithAudio = inWithAudio; mRestart =
true;}
86 inline void CheckFor4kInput (
const bool inCheckFor4K) {mCheckFor4K = inCheckFor4K;}
94 inline bool GetDeinterlaceNonProgressiveVideo (
void)
const {
return mDeinterlace;}
110 void newFrame (
const QImage &inImage,
const bool inClear);
125 void changeCaptionChannel (
int id);
129 virtual void run (
void);
165 bool mbFixedReference;
177 bool mFormatIsProgressive;
183 bool mDoMultiChannel;
186 #if (QT_VERSION < QT_VERSION_CHECK(6, 0, 0))
187 QAudioOutput * mAudioOutput;
189 QAudioSink * mAudioOutput;
191 QAudioFormat mFormat;
192 QIODevice * mAudioDevice;
196 std::string mTimeCode;
198 #if defined (INCLUDE_AJACC)
201 ushort mScreenBuffer [15][32];
205 #endif // defined (INCLUDE_AJACC)
210 #endif // NTV2FRAMEGRABBER_H
UWord GetDeviceIndex(void) const
void SetDeinterlaceNonProgressiveVideo(const bool inDeinterlace)
Enables or disables deinterlacing of non-progressive video.
Describes the horizontal and vertical size dimensions of a raster, bitmap, frame or image.
Declares common types used in the ajabase library.
bool SetupInput(void)
Configures my AJA device for capture.
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
Declares the CNTV2Task class (deprecated).
NTV2VideoFormat GetVideoFormatFromInputSource(void)
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
void CheckFor4kInput(const bool inCheckFor4K)
Enables or disables checking for 4K/UHD video (on devices that supported 4K/UHD).
bool CheckForValidInput(void)
This class is used to respond to dynamic events that occur during CEA-608 caption decoding.
Declares the AJAProcess class.
void SetWithAudio(const bool inWithAudio)
Enables or disables host audio playback.
void SetupAudio(void)
Performs audio configuration.
void captionScreenChanged(const ushort *pInScreen)
This is signaled (called) when my caption screen buffer changes.
Declares the CNTV2Card class.
NTV2TCIndex
These enum values are indexes into the capture/playout AutoCirculate timecode arrays.
Declares the CNTV2CaptionDecoder708 class.
Enumerations for controlling NTV2 devices.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
NTV2FrameGrabber(QObject *pInParentObject=NULL)
Constructs me.
I interrogate and control an AJA video/audio capture/playout device.
void newStatusString(const QString &inStatus)
This is signaled (called) when my status string changes.
void OutputAudio(ULWord *pInOutAudioBuffer, const ULWord inNumValidBytes)
Writes audio samples for channels 1 and 2 that are in the given audio buffer to my QAudioOutput devic...
NTV2InputSource
Identifies a specific video input source.
void ClearCaptionBuffer(const bool inSignalClients=false)
virtual void run(void)
My thread function.
A QThread that captures audio/video from NTV2-compatible AJA devices and uses Qt signals to emit ARGB...
void GrabCaptions(void)
Performs caption data extraction & decoding.
void SetInputSource(const NTV2InputSource inInputSource)
Sets the input to be used for capture on the AJA device being used.
void newFrame(const QImage &inImage, const bool inClear)
This is signaled (called) when a new frame has been captured and is available for display.
bool IsInput3Gb(const NTV2InputSource inputSource)
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
Declares the CRP188 class. See SMPTE RP188 standard for details.
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
void StopAutoCirculate(void)
Stops capturing.
void SetDeviceIndex(const UWord inDeviceIndex)
Sets the AJA device to be used for capture.
void SetFixedReference(bool fixed)
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
void SetTimeCodeSource(const NTV2TCIndex inTCSource)
Declares the CNTV2CaptionDecoder608 class.
NTV2LHIHDMIColorSpace GetColorSpaceFromInputSource(void)
virtual ~NTV2FrameGrabber()
My destructor.