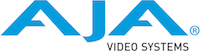 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
7 #ifndef __NTV2_CEA608_DECODEXDSCHANNEL_
8 #define __NTV2_CEA608_DECODEXDSCHANNEL_
18 #elif defined (AJAMac)
19 #pragma GCC diagnostic ignored "-Wunused-private-field"
123 virtual void Init (
void);
124 virtual void Reset (
void);
159 UByte mProgramIDNumberData [4];
160 int mProgramIDNumberCount;
162 UByte mLengthTimeInShowData [6];
163 int mLengthTimeInShowCount;
165 char mProgramNameStr [33];
167 UByte mProgramTypeData [2];
168 int mProgramTypeCount;
170 UByte mContentAdvisoryData [2];
171 int mContentAdvisoryCount;
173 UByte mAudioServicesData [2];
174 int mAudioServicesCount;
176 UByte mCaptioningServicesData [8];
177 int mCaptioningServicesCount;
179 UByte mCopyRedistributionData [2];
180 int mCopyRedistributionCount;
182 UByte mCompositePacket1Data [33];
183 int mCompositePacket1Count;
185 UByte mCompositePacket2Data [33];
186 int mCompositePacket2Count;
188 char mProgramDescriptionRow1Str [33];
189 char mProgramDescriptionRow2Str [33];
190 char mProgramDescriptionRow3Str [33];
191 char mProgramDescriptionRow4Str [33];
192 char mProgramDescriptionRow5Str [33];
193 char mProgramDescriptionRow6Str [33];
194 char mProgramDescriptionRow7Str [33];
195 char mProgramDescriptionRow8Str [33];
198 char mNetworkNameStr [33];
200 UByte mCallLettersData [6];
201 int mCallLettersCount;
203 UByte mTapeDelayData [2];
206 UByte mTransmissionSignalIDData [4];
207 int mTransmissionSignalIDCount;
210 UByte mTimeOfDayData [6];
213 UByte mImpulseCaptureIDData [6];
214 int mImpulseCaptureIDCount;
216 UByte mSupplementalDataLocationData [32];
217 int mSupplementalDataLocationCount;
219 UByte mLocalTimeZoneData [2];
220 int mLocalTimeZoneCount;
222 UByte mOutOfBandChannelNumberData [2];
223 int mOutOfBandChannelNumberCount;
225 UByte mChannelMapPointerData [2];
226 int mChannelMapPointerCount;
228 UByte mChannelMapHeaderData [4];
229 int mChannelMapHeaderCount;
231 UByte mChannelMapData [10];
232 int mChannelMapCount;
234 char mNWSCodeStr [33];
235 char mNWSMessageStr [33];
237 int mDebugPrintOffset;
240 void * mpSubscriberData;
244 #endif // __NTV2_CEA608_DECODEXDSCHANNEL_
@ NTV2_CC608_XDSProgramDescRow8Type
@ NTV2_CC608_XDSChannelMapHeaderType
@ NTV2_CC608_XDSUnknownClass
Declares several data types used with 608/SD captioning.
@ NTV2_CC608_XDSChannelMapPointerType
@ NTV2_CC608_XDSProgramDescRow3Type
@ NTV2_CC608_XDSProgramDescRow1Type
@ NTV2_CC608_XDSCompositePacket1Type
@ NTV2_CC608_XDSTransmissionSignalIDType
@ NTV2_CC608_XDSCaptionServicesType
AJARefPtr< CNTV2XDSDecodeChannel608 > CNTV2XDSDecodeChannel608Ptr
@ NTV2_CC608_XDSImpulseCaptureIDType
@ NTV2_CC608_XDSCompositePacket2Type
@ NTV2_CC608_XDSLocalTimeZoneType
@ NTV2_CC608_XDSNWSMessage
@ NTV2_CC608_XDSTimeOfDayType
@ NTV2_CC608_XDSLengthTimeInShowType
@ NTV2_CC608_XDSNetworkNameType
@ NTV2_CC608_XDSUnknownType
@ NTV2_CC608_XDSContentAdvisoryType
@ NTV2_CC608_XDSProgramDescRow5Type
@ NTV2_CC608_XDSProgramNameType
@ NTV2_CC608_XDSProgramIDNumberType
@ NTV2_CC608_XDSOutOfBandChannelType
@ NTV2_CC608_XDSNumClasses
void() NTV2Caption608Changed(void *pInstance, const NTV2Caption608ChangeInfo &inChangeInfo)
This callback is used to respond to dynamic events that occur during CEA-608 caption decoding.
@ NTV2_CC608_XDSChannelClass
@ NTV2_CC608_XDSAudioServicesType
@ NTV2_CC608_XDSChannelMapType
NTV2Line21Channel
The CEA-608 caption channels: CC1 thru CC4, TX1 thru TX4, plus XDS.
@ NTV2_CC608_XDSSupplementalDataLocationType
@ NTV2_CC608_XDSProgramDescRow7Type
@ NTV2_CC608_XDSReservedClass
@ NTV2_CC608_XDSProgramTypeType
@ NTV2_CC608_XDSProgramDescRow6Type
@ NTV2_CC608_XDSProgramDescRow2Type
@ NTV2_CC608_XDSFutureClass
@ NTV2_CC608_XDSPrivateDataClass
@ NTV2_CC608_XDSCopyRedistributionType
NTV2Line21Field
The two CEA-608 interlace fields.
@ NTV2_CC608_XDSPublicServiceClass
Defines the AJARefPtr template class.
@ NTV2_CC608_XDSCurrentClass
@ NTV2_CC608_XDSTapeDelayType
Declares the NTV2CaptionLogMask, and the CNTV2CaptionLogConfig class.
@ NTV2_CC608_XDSProgramDescRow4Type
@ NTV2_CC608_XDSCallLettersType
@ NTV2_CC608_XDSMiscClass