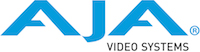 |
AJA NTV2 SDK
17.5.0.1242
NTV2 SDK 17.5.0.1242
|
Go to the documentation of this file.
9 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
13 #if defined(AJA_WINDOWS)
15 #elif defined(AJA_LINUX)
17 #elif defined(AJA_MAC)
19 #elif defined(AJA_BAREMETAL)
27 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
28 mpMutex =
new recursive_timed_mutex;
39 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
40 mpMutex =
new recursive_timed_mutex;
57 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
71 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
74 bool success = mpMutex->try_lock_for(std::chrono::milliseconds(timeout));
83 return mpImpl->
Lock(timeout);
91 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
Declares the AJALockImpl class.
#define LOCK_TIME_INFINITE
Declares the AJALockImpl class.
Declares the AJALock class.
AJALock(const char *pName=NULL)
virtual AJAStatus Lock(uint32_t timeout=0xffffffff)
Declares the AJALockImpl class.
AJAStatus Lock(uint32_t uTimeout=0xffffffff)
virtual AJALock & operator=(const AJALock &inLock)
AJAAutoLock(AJALock *pLock=NULL)
virtual AJAStatus Unlock()