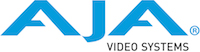 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
7 #ifndef __NTV2_CEA708_ENCODER_
8 #define __NTV2_CEA708_ENCODER_
27 #define ServiceBlockHeaderSize(svcNum) (svcNum <= 6 ? 1 : 2)
555 inline bool IsValid (
void)
const {
return row >= 0 && row <= 14 && column >= 0 &&
column <= 41;}
604 virtual void Reset (
void);
607 virtual void InitCaptionChannelPacket ();
608 virtual bool SetCaptionChannelPacket (
const UBytePtr pInData,
const size_t numBytes);
612 virtual bool SetCaptionChannelPacketSize (
size_t packetSize);
614 virtual bool MakeCaptionChannelPacketHeader (
const size_t index,
size_t packetSize,
size_t & outNewIndex);
615 virtual bool MakeNullServiceBlockHeader (
size_t index,
size_t & outNewIndex);
616 virtual bool MakeServiceBlockHeader (
const size_t index,
int serviceNum,
const size_t blockSize,
size_t & outNewIndex);
617 virtual bool MakeServiceBlockHeader (
const size_t index,
int serviceNum,
const size_t blockSize);
618 virtual bool MakeServiceBlockCharData (
const size_t index,
UByte data,
size_t & outNewIndex);
620 virtual bool MakeDefineWindowCommand (
const size_t index,
int windowID,
const CC708WindowParms & inParms,
size_t & outNewIndex);
621 virtual bool MakeClearWindowsCommand (
const size_t index,
UByte windowMap,
size_t & outNewIndex);
622 virtual bool MakeDeleteWindowsCommand (
const size_t index,
UByte windowMap,
size_t & outNewIndex);
623 virtual bool MakeDisplayWindowsCommand (
const size_t index,
UByte windowMap,
size_t & outNewIndex);
624 virtual bool MakeHideWindowsCommand (
const size_t index,
UByte windowMap,
size_t & outNewIndex);
625 virtual bool MakeToggleWindowsCommand (
const size_t index,
UByte windowMap,
size_t & outNewIndex);
626 virtual bool MakeSetCurrentWindowCommand (
const size_t index,
const int windowID,
size_t & outNewIndex);
627 virtual bool MakeSetWindowAttributesCommand (
const size_t index,
const CC708WindowAttr & inAttr,
size_t & outNewIndex);
629 virtual bool MakeSetPenAttributesCommand (
const size_t index,
const CC708PenAttr & inAttr,
size_t & outNewIndex);
630 virtual bool MakeSetPenColorCommand (
const size_t index,
const CC708PenColor & inColor,
size_t & outNewIndex);
631 virtual bool MakeSetPenLocationCommand (
const size_t index,
const CC708PenLocation & inLoc,
size_t & outNewIndex);
633 virtual bool MakeDelayCommand (
const size_t index,
const UByte delay,
size_t & outNewIndex);
634 virtual bool MakeDelayCancelCommand (
const size_t index,
size_t & outNewIndex);
635 virtual bool MakeResetCommand (
const size_t index,
size_t & outNewIndex);
644 virtual bool Clear608CaptionData (
void);
652 virtual bool Set608CaptionData (
const CaptionData & inCC608Data);
661 virtual bool Set608CaptionData (
const NTV2Line21Field inField,
const UByte inChar1,
const UByte inChar2,
const bool inGotData);
697 virtual bool MakeSMPTE334AncPacketFromCDP (
const UBytePtr pInCDP,
const size_t inCDPLength,
UWordPtr & outAncPacketData,
size_t & outSize);
706 virtual bool MakeSMPTE334AncPacketFromCDP (
const UBytePtr pInCDP,
const size_t inCDPLength);
708 virtual bool SetServiceInfoActive (
int svcIndex,
bool bActive);
724 virtual bool InsertSMPTE334AncPacketInVideoFrame (
void * pFrameBuffer,
727 const ULWord inVancLineNumber,
728 const ULWord inWordOffset = 1)
const;
732 virtual inline void Set608TestIDMode (
const bool inEnableTestIDMode) { mFlip608Characters = inEnableTestIDMode; }
741 virtual bool InsertSMPTE334AncHeader (
size_t & inOutAncIndex,
const UByte inDataCount);
742 virtual bool InsertSMPTE334AncFooter (
size_t & inOutAncIndex,
const size_t inAncStartIndex);
743 virtual bool InsertCDPHeader (
size_t & inOutAncIndex,
const NTV2FrameRate inFrameRate,
const int cdpSeqNum);
744 virtual bool InsertCDPFooter (
size_t & inOutAncIndex,
const size_t cdpStartIndex,
const int cdpSeqNum);
746 virtual bool InsertCDPDataTriplet (
size_t & inOutAncIndex,
const bool ccValid,
const int ccType,
const UByte data1,
const UByte data2);
747 virtual bool InsertCDPServiceInfo (
size_t & inOutAncIndex);
756 static bool ConvertFrameRate (
const NTV2FrameRate inNTV2FrameRate,
size_t & outConverted,
size_t & outNumCCPackets,
size_t & outNum608Triplets);
764 size_t mPacketDataSize;
765 int mPacketSequenceNum;
768 UWord mCDPSequenceNum;
770 int mNumServiceInfoPerCDP;
771 bool mFlip608Characters;
775 #endif // __NTV2_CEA708_ENCODER_
@ NTV2_CC708DispEffectWipe
@ NTV2_CC708ScrollDirRtoL
@ NTV2_CC708CDPHeader_CCDataPresent
const size_t NTV2_CC708MaxAncSize
@ NTV2_CC708BorderTypeMax
@ NTV2_CC708PenEdgeTypeMax
const CC708Color NTV2_CC708WhiteColor
@ NTV2_CC708DispEffectMin
@ NTV2_CC708BorderTypeUniform
Declares several data types used with 608/SD captioning.
const size_t NTV2_CC708_MaxServiceBlockSize
@ NTV2_CC708TextTagDialog
@ NTV2_CC708PenEdgeTypeNone
@ NTV2_CC708DispEffectSnap
@ NTV2_CC708TextTagExpletive
@ NTV2_CC708TextTagElectronic
@ NTV2_CC708CDPFrameRate23p98
struct NTV2_CC708CDPDataSection * NTV2_CC708CDPDataSectionPtr
@ NTV2_CC708TextTagTextNoDisplay
struct NTV2_CC708CDPHeader * NTV2_CC708CDPHeaderPtr
@ NTV2_CC708CDPHeader_Reserved
@ NTV2_CC708PenOffsetSuperscript
@ NTV2_CC708CCTypeNTSCField2
const size_t NTV2_CC708_MaxCaptionChannelPacketSize
Declares the CNTV2Caption708ServiceInfo class.
CC708WindowParms()
NTV2_CC708PenStyleID enums.
const CC708Color NTV2_CC708MagentaColor(NTV2_CC708ColorMax, NTV2_CC708ColorMin, NTV2_CC708ColorMax)
@ NTV2_CC708CDPHeader_CaptionServiceActive
@ NTV2_CC708TextTagLyrics
@ NTV2_CC708BorderTypeShdwLeft
@ NTV2_CC708CDPFrameRate30
@ NTV2_CC708EffectDirRtoL
struct NTV2_CC708CDP * NTV2_CC708CDPPtr
struct CC708PenAttr CC708PenAttr
@ NTV2_CC708ScrollDirTtoB
int anchorPt
NTV2_CC708WindowPriority enums.
virtual void Set608TestIDMode(const bool inEnableTestIDMode)
const bool NTV2_CC708RelativePos
@ NTV2_CC708WindowAnchorPointLowerMiddle
struct CC708Color CC708Color
@ NTV2_CC708CDPFrameRate24
NTV2_CC708CDPTimecodeSection timecode_section
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
@ NTV2_CC708CDPHeader_SvcInfoPresent
@ NTV2_CC708CDPFrameRate59p94
const bool NTV2_CC708WordWrap
@ NTV2_CC708PenOffsetSubscript
const bool NTV2_CC708AbsolutePos
@ NTV2_CC708WindowAnchorPointUpperLeft
@ NTV2_CC708FontStyleSmallCaps
@ NTV2_CC708PenEdgeTypeMin
virtual UBytePtr GetCaptionChannelPacket(void)
@ NTV2_CC708_CDPServiceInfoId
@ NTV2_CC708TextTagVoiceDesc
@ NTV2_CC708TextTagReserved2
const size_t NTV2_CC708MaxPktSize
NTV2_CC708CDPDataTriplet cc_data[32]
const int NTV2_CC708ScreenCellWidth16x9
@ NTV2_CC708EffectDirTtoB
I am a container for all of the CEA-708 "Service Information" that a decoder or encoder needs to keep...
struct NTV2_CC708CDPHeader NTV2_CC708CDPHeader
NTV2FrameRate
Identifies a particular video frame rate.
virtual const UWord * GetSMPTE334Data(void) const
@ NTV2_CC708WindowStyleIDMax
@ NTV2_CC708WindowAnchorPointMin
@ NTV2_CC708PenEdgeTypeRightDropShadow
@ NTV2_CC708WindowStyleIDMin
std::ostream & operator<<(std::ostream &inOutStream, const CC708Color &inData)
@ NTV2_CC708PenEdgeTypeUniform
@ NTV2_CC708TextTagReserved1
virtual size_t GetSMPTE334Size(void) const
@ NTV2_CC708BorderTypeNone
const bool NTV2_CC708Underline(true)
NTV2_CC708CDPDataSection ccdata_section
const CC708Color NTV2_CC708CyanColor(NTV2_CC708ColorMin, NTV2_CC708ColorMax, NTV2_CC708ColorMax)
const int NTV2_CC708ScreenCellHeight
@ NTV2_CC708CDPHeader_SvcInfoComplete
@ NTV2_CC708DispEffectFade
const bool NTV2_CC708Visible
@ NTV2_CC708FontStylePropSanSerif
@ NTV2_CC708TextTagAudibleTrans
@ NTV2_CC708CCTypeDTVCCStart
@ NTV2_CC708TextTagVoiceover
CC708PenLocation(const int inRow=0, const int inColumn=0)
@ NTV2_CC708PenOffsetNormal
NTV2_CC708CDPServiceInfoSection ccsvcinfo_section
const bool NTV2_CC708NotVisible
const CC708Color NTV2_CC708BlueColor(NTV2_CC708ColorMin, NTV2_CC708ColorMin, NTV2_CC708ColorMax)
std::vector< uint16_t > UWordSequence
An ordered sequence of UWord (uint16_t) values.
int penStyleID
NTV2_CC708WindowStyleID enums.
UByte time_code_section_id
CC708PenAttr()
Default constructor.
const bool NTV2_CC708NoWordWrap
virtual NTV2CaptionLogMask SetLogMask(const NTV2CaptionLogMask inLogMask)
Specifies what, if any, debug information I will write to my log stream.
@ NTV2_CC708TextTagReserved3
@ NTV2_CC708TextTagMusicScore
@ NTV2_CC708BorderTypeShdwRight
@ NTV2_CC708ScrollDirBtoT
const int NTV2_CC708DefaultOpacity(NTV2_CC708OpacitySolid)
std::string NTV2_CC708OpacityToString(const NTV2_CC708Opacity inOpacity, const bool inCompact=false)
struct CC708WindowParms CC708WindowParms
struct NTV2_CC708CDPFooter NTV2_CC708CDPFooter
@ NTV2_CC708CDPHeader_TimeCodePresent
@ NTV2_CC708PenSizeStandard
@ NTV2_CC708CDPHeader_SvcInfoStart
@ NTV2_CC708OpacityOpaque
struct CC708PenLocation CC708PenLocation
struct NTV2_CC708CDP NTV2_CC708CDP
@ NTV2_CC708PenEdgeTypeRaised
@ NTV2_CC708FontStyleCursive
struct NTV2_CC708CDPFooter * NTV2_CC708CDPFooterPtr
@ NTV2_CC708WindowPriorityMin
bool relativePos
NTV2_CC708WindowAnchorPoint enums.
@ NTV2_CC708BorderTypeRaised
@ NTV2_CC708_CDPHeaderId1
@ NTV2_CC708FontStylePropSerif
@ NTV2_CC708WindowPriorityMax
@ NTV2_CC708TextTagSecLanguage
@ NTV2_CC708WindowAnchorPointUpperRight
@ NTV2_CC708CDPFrameRate60
@ NTV2_CC708CDPFrameRate29p97
const CC708Color NTV2_CC708RedColor(NTV2_CC708ColorMax, NTV2_CC708ColorMin, NTV2_CC708ColorMin)
@ NTV2_CC708BorderTypeMin
@ NTV2_CC708PenEdgeTypeDepressed
@ NTV2_CC708BorderTypeDepressed
@ NTV2_CC708CDPFrameRate50
struct NTV2_CC708CDPTimecodeSection * NTV2_CC708CDPTimecodeSectionPtr
@ NTV2_CC708TextTagSoundEfx
@ NTV2_CC708WindowAnchorPointCenterMiddle
@ NTV2_CC708WindowAnchorPointLowerRight
const CC708Color NTV2_CC708GreenColor(NTV2_CC708ColorMin, NTV2_CC708ColorMax, NTV2_CC708ColorMin)
const bool NTV2_CC708NoLock
struct NTV2_CC708CDPServiceInfoSection * NTV2_CC708CDPServiceInfoSectionPtr
@ NTV2_CC708CCTypeNTSCField1
bool visible
NTV2_CC708NoLock/NTV2_CC708Lock.
bool colLock
NTV2_CC708NoLock/NTV2_CC708Lock.
const bool NTV2_CC708Lock
struct NTV2_CC708CDPTimecodeSection NTV2_CC708CDPTimecodeSection
@ NTV2_CC708WindowAnchorPointCenterRight
const bool NTV2_CC708NoUnderline(false)
@ NTV2_CC708FontStyleUndefined
@ NTV2_CC708FontStyleMonoSansSerif
const bool NTV2_CC708Italics(true)
@ NTV2_CC708TextTagSpeaker
int colCount
number of rows - 1 (e.g. '0' = 1 row)
@ NTV2_CC708ScrollDirLtoR
@ NTV2_CC708DispEffectMax
struct NTV2_CC708CDPDataTriplet NTV2_CC708CDPDataTriplet
@ NTV2_CC708WindowAnchorPointMax
int rowCount
0 - 255 (absolute position) or 0 - 99 (relative position)
@ NTV2_CC708TextTagSubtitleTrans
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
@ NTV2_CC708FontStyleMonoSerif
@ NTV2_CC708PenEdgeTypeLeftDropShadow
@ NTV2_CC708OpacityTransparent
struct NTV2_CC708CDPDataSection NTV2_CC708CDPDataSection
@ NTV2_CC708EffectDirLtoR
bool rowLock
number of columns - 1 (e.g. '0' = 1 column)
@ NTV2_CC708FontStyleCasual
uint64_t NTV2CaptionLogMask
Selectors to control what information is logged.
int anchorV
NTV2_CC708AbsolutePos/NTV2_CC708RelativePos.
struct NTV2_CC708CDPServiceInfoSection NTV2_CC708CDPServiceInfoSection
@ NTV2_CC708CDPHeader_SvcInfoChange
@ NTV2_CC708_CDPHeaderId2
virtual const UByte * GetCaptionChannelPacket(void) const
struct CC708WindowAttr CC708WindowAttr
const bool NTV2_CC708NoItalics(false)
const CC708Color NTV2_CC708YellowColor(NTV2_CC708ColorMax, NTV2_CC708ColorMax, NTV2_CC708ColorMin)
@ NTV2_CC708WindowAnchorPointLowerLeft
struct CC708PenColor CC708PenColor
@ NTV2_CC708OpacityTranslucent
@ NTV2_CC708WindowAnchorPointCenterLeft
@ NTV2_CC708JustifyCenter
NTV2Line21Field
The two CEA-608 interlace fields.
@ NTV2_CC708WindowAnchorPointUpperMiddle
This structure encapsulates all possible CEA-608 caption data bytes that may be associated with a giv...
int anchorH
0 - 127 (absolute position) or 0 - 99 (relative position)
NTV2_CC708ServiceInfo svc_info[16]
virtual size_t GetCaptionChannelPacketSize(void) const
@ NTV2_CC708PenStyleIDMax
@ NTV2_CC708CCTypeDTVCCData
NTV2_CC708CDPFooter cdp_footer
Defines the AJARefPtr template class.
NTV2_CC708CDPHeader cdp_header
int windowStyleID
NTV2_CC708Visible/NTV2_CC708NotVisible.
struct NTV2_CC708CDPDataTriplet NTV2_CC708CDPDataTripletPtr
@ NTV2_CC708EffectDirBtoT
@ NTV2_CC708CDPFrameRate25
const int NTV2_CC708ScreenCellWidth4x3
const CC708Color NTV2_CC708BlackColor(NTV2_CC708ColorMin, NTV2_CC708ColorMin, NTV2_CC708ColorMin)
@ NTV2_CC708PenStyleIDMin
@ NTV2_CC708_CDPTimecodeId
AJARefPtr< CNTV2CaptionEncoder708 > CNTV2CaptionEncoder708Ptr