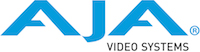 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
8 #ifndef AJA_DPXFILEIO_H
9 #define AJA_DPXFILEIO_H
75 const uint32_t inBufferSize,
117 const uint32_t inBufferSize,
118 const uint32_t & inIndex)
const;
132 uint32_t mCurrentIndex;
133 std::vector<std::string> mFileList;
137 #endif // AJA_DPXFILEIO_H
AJA_EXPORT std::string GetPath() const
Returns the current path to the DPX files to be read.
Declares common types used in the ajabase library.
AJA_EXPORT uint32_t GetIndex() const
Returns the index in the file list of the next file to be read.
AJA_EXPORT AJAStatus SetIndex(const uint32_t &index)
Specifies the index in the file list of the next file to be read.
AJA_EXPORT void SetPauseMode(bool mode)
Specifies the setting of the pause control. If true, pause mode will take effect. If false,...
AJA_EXPORT void SetLoopMode(bool mode)
Specifies the setting of the loop play control. If true, the last file of a sequence is followed by t...
AJA_EXPORT uint32_t GetFileCount() const
Returns the number of DPX files in the location specified by the path.
AJA_EXPORT AJAStatus Read(const uint32_t inIndex)
Read only the header from the specified file in the DPX sequence.
void SetFileList(std::vector< std::string > &list)
Specifies an ordered list of DPX files to read.
AJA_EXPORT AJAStatus SetPath(const std::string &inPath)
Change the path to the DPX files to be read.
AJA_EXPORT bool GetLoopMode() const
Returns the current setting of the loop play control.
AJA_EXPORT std::vector< std::string > & GetFileList()
Returns the list of DPX files that were found at the destination.
Declaration of DpxHdr class adapted from STwo's dpx file I/O.
AJA_EXPORT bool GetPauseMode() const
Returns the current setting of the pause control.
AJA_EXPORT AJAStatus Write(const uint8_t &inBuffer, const uint32_t inBufferSize, const uint32_t &inIndex) const
Write a DPX file.
AJA_EXPORT AJADPXFileIO()
Constructs a DPX file IO object.
virtual AJA_EXPORT ~AJADPXFileIO()