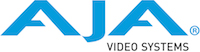 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
9 #ifndef _NTV2ENCODEHEVCFILEAC_H
10 #define _NTV2ENCODEHEVCFILEAC_H
28 #define VIDEO_RING_SIZE 60
29 #define AUDIO_RING_SIZE (3*VIDEO_RING_SIZE)
67 const bool inQuadMode =
false,
68 const uint32_t inAudioChannels = 0,
69 const bool inTimeCodeBurn =
false,
70 const bool inInfoData =
false,
71 const uint32_t inMaxFrames = 0xffffffff);
89 virtual void Quit (
void);
263 const std::string mDeviceSpecifier;
278 uint32_t mNumAudioChannels;
279 uint32_t mFileAudioChannels;
283 bool mLastFrameInput;
286 bool mLastFrameVideo;
287 bool mLastFrameAudio;
290 uint32_t mVideoBufferSize;
291 uint32_t mPicInfoBufferSize;
292 uint32_t mEncInfoBufferSize;
293 uint32_t mAudioBufferSize;
307 uint32_t mVideoInputFrameCount;
308 uint32_t mVideoProcessFrameCount;
309 uint32_t mCodecRawFrameCount;
310 uint32_t mCodecHevcFrameCount;
311 uint32_t mVideoFileFrameCount;
312 uint32_t mAudioFileFrameCount;
320 #endif // _NTV2ENCODEHEVCFILEAC_H
virtual void StartCodecRawThread(void)
Start the codec raw thread.
@ NTV2_FBF_10BIT_YCBCR_420PL2
10-Bit 4:2:0 2-Plane YCbCr
virtual void CodecHevcWorker(void)
Repeatedly transfers hevc frames from the codec and adds them to the hevc ring.
virtual void StartVideoProcessThread(void)
Start the video process thread.
Declares device capability functions.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
This file contains some structures, constants, classes and functions that are used in some of the hev...
static void VideoFileThreadStatic(AJAThread *pThread, void *pContext)
This is the video file writer thread's static callback function that gets called when the thread star...
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing video.
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
static void VideoProcessThreadStatic(AJAThread *pThread, void *pContext)
This is the video process thread's static callback function that gets called when the thread starts....
Declares the AJATimeCode class.
@ NTV2_CHANNEL1
Specifies channel or FrameStore 1 (or the first item).
virtual void SetupAutoCirculate(void)
Initializes AutoCirculate.
virtual M31VideoPreset GetCodecPreset(void)
Get the codec preset.
virtual AJAStatus ProcessVideoFrame(AVHevcDataBuffer *pSrcFrame, AVHevcDataBuffer *pDstFrame, uint32_t frameNumber)
Default do-nothing function for processing the captured frames.
virtual void CodecRawWorker(void)
Repeatedly removes video frames from the raw video ring and transfers them to the codec.
static void CodecRawThreadStatic(AJAThread *pThread, void *pContext)
This is the codec raw thread's static callback function that gets called when the thread starts....
Declares the AJATimeCodeBurn class.
virtual void GetStatus(AVHevcStatus &outStatus)
Provides status information about my input (capture) process.
virtual void StartVideoFileThread(void)
Start the video file writer thread.
Declares the CNTV2DeviceScanner class.
virtual AJAStatus Run(void)
Runs me.
Enumerations for controlling NTV2 devices.
virtual void VideoProcessWorker(void)
Repeatedly removes video frames from the video input ring, calls a custom video process method and ad...
static void CodecHevcThreadStatic(AJAThread *pThread, void *pContext)
This is the codec hevc thread's static callback function that gets called when the thread starts....
I interrogate and control an AJA video/audio capture/playout device.
virtual void StartVideoInputThread(void)
Start the video input thread.
@ M31_FILE_1280X720_420_8_5994p
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing audio.
static void VideoInputThreadStatic(AJAThread *pThread, void *pContext)
This is the video input thread's static callback function that gets called when the thread starts....
NTV2InputSource
Identifies a specific video input source.
virtual void RouteInputSignal(void)
Sets up device routing for capture.
Declares the enumeration constants used in the ajabase library.
virtual void StartCodecHevcThread(void)
Start the codec hevc thread.
static void AudioFileThreadStatic(AJAThread *pThread, void *pContext)
This is the audio file writer thread's static callback function that gets called when the thread star...
Enumerations for controlling NTV2 devices with m31 HEVC encoders.
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
virtual ~NTV2EncodeHEVCFileAc()
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
virtual void StartAudioFileThread(void)
Start the audio file writer thread.
virtual void AudioFileWorker(void)
Repeatedly removes audio samples from the audio input ring and writes them to the audio output file.
virtual void VideoFileWorker(void)
Repeatedly removes hevc frame from the hevc ring and writes them to the hevc output file.
virtual void VideoInputWorker(void)
Repeatedly captures video frames using AutoCirculate and add them to the video input ring.
Declaration of AJACircularBuffer template class.
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
This structure encapsulates the video and audio buffers used by the HEVC demo applications....
Declares the AJAThread class.
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
NTV2EncodeHEVCFileAc(const std::string inDeviceSpecifier="0", const NTV2Channel inChannel=NTV2_CHANNEL1, const M31VideoPreset inM31Preset=M31_FILE_1280X720_420_8_5994p, const NTV2FrameBufferFormat inPixelFormat=NTV2_FBF_10BIT_YCBCR_420PL2, const bool inQuadMode=(0), const uint32_t inAudioChannels=0, const bool inTimeCodeBurn=(0), const bool inInfoData=(0), const uint32_t inMaxFrames=0xffffffff)
Constructs me using the given settings.
Utility class for timecodes.
Declares the AJATimeBase class.
virtual void Quit(void)
Gracefully stops me from running.