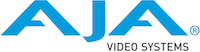 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
81 if (mCurrentIndex >= mFileCount)
85 string fileName = mFileList [mCurrentIndex];
104 const uint32_t bufferSize,
117 if (mCurrentIndex >= mFileCount)
121 string fileName = mFileList [mCurrentIndex];
157 index = mCurrentIndex;
164 if (mLoopMode && (mCurrentIndex + 1 >= mFileCount))
188 if (index && (index > mFileCount - 1))
191 mCurrentIndex = index;
224 mFileCount = uint32_t(mFileList.size());
234 const uint32_t bufferSize,
235 const uint32_t & index)
const
246 char asciiSequence[9];
247 ajasnprintf (asciiSequence, 9,
"%.8d", index);
249 fileName = mPath +
"/" + asciiSequence +
".DPX";
258 uint32_t bytesWritten = file.
Write ((
const uint8_t*)&
GetHdr(), uint32_t(headerSize));
259 if (bytesWritten != headerSize)
265 bytesWritten = file.
Write ((uint8_t*)&buffer, bufferSize);
266 if (bytesWritten != bufferSize)
uint32_t Write(const uint8_t *pBuffer, const uint32_t length) const
AJA_EXPORT std::string GetPath() const
Returns the current path to the DPX files to be read.
AJA_EXPORT uint32_t GetIndex() const
Returns the index in the file list of the next file to be read.
AJA_EXPORT AJAStatus SetIndex(const uint32_t &index)
Specifies the index in the file list of the next file to be read.
const DPX_header_t & GetHdr(void) const
AJA_EXPORT void SetPauseMode(bool mode)
Specifies the setting of the pause control. If true, pause mode will take effect. If false,...
size_t get_ii_image_size()
AJA_EXPORT void SetLoopMode(bool mode)
Specifies the setting of the loop play control. If true, the last file of a sequence is followed by t...
AJAStatus Open(const std::string &fileName, const int flags, const int properties)
AJA_EXPORT uint32_t GetFileCount() const
Returns the number of DPX files in the location specified by the path.
AJAStatus Seek(const int64_t distance, const AJAFileSetFlag flag) const
Declaration of the AJADPXFileIO class, for low level file I/O.
AJA_EXPORT AJAStatus Read(const uint32_t inIndex)
Read only the header from the specified file in the DPX sequence.
void SetFileList(std::vector< std::string > &list)
Specifies an ordered list of DPX files to read.
size_t get_fi_image_offset() const
size_t GetHdrSize(void) const
AJA_EXPORT AJAStatus SetPath(const std::string &inPath)
Change the path to the DPX files to be read.
uint32_t Read(uint8_t *pBuffer, const uint32_t length)
Declares the AJAFileIO class.
AJA_EXPORT bool GetLoopMode() const
Returns the current setting of the loop play control.
AJA_EXPORT std::vector< std::string > & GetFileList()
Returns the list of DPX files that were found at the destination.
static AJAStatus ReadDirectory(const std::string &directory, const std::string &filePattern, std::vector< std::string > &fileContainer)
AJA_EXPORT bool GetPauseMode() const
Returns the current setting of the pause control.
AJA_EXPORT AJAStatus Write(const uint8_t &inBuffer, const uint32_t inBufferSize, const uint32_t &inIndex) const
Write a DPX file.
AJA_EXPORT AJADPXFileIO()
Constructs a DPX file IO object.
virtual AJA_EXPORT ~AJADPXFileIO()