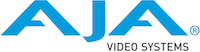 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
25 inline SRTWindow (uint32_t inX1 = 0, uint32_t inY1 = 0,
26 uint32_t inX2 = 0, uint32_t inY2 = 0)
27 {
set(inX1, inY1, inX2, inY2);}
29 inline uint32_t
top (
void)
const {
return mY1;}
30 inline uint32_t
left (
void)
const {
return mX1;}
31 inline uint32_t
bottom (
void)
const {
return mY2;}
32 inline uint32_t
right (
void)
const {
return mX2;}
37 inline bool operator == (
const SRTWindow & inRHS)
const {
return this == &inRHS || (mX1 == inRHS.mX1 && mX2 == inRHS.mX2 && mY1 == inRHS.mY1 && mY2 == inRHS.mY2);}
40 std::ostream &
print (std::ostream & oss)
const;
43 inline void clear (
void) {mX1 = mY1 = mX2 = mY2 = 0;}
50 inline SRTWindow &
set (uint32_t inX1, uint32_t inY1, uint32_t inX2, uint32_t inY2)
53 uint32_t mX1, mX2, mY1, mY2;
65 const SRTDuration inDuration = 0,
const std::string & inRawCaption = std::string(),
66 const size_t inStartLineNum = 0,
const size_t inEndLineNum = 0)
67 {
set(inSeqNum, inStartTime, inDuration, inRawCaption, inStartLineNum, inEndLineNum);}
69 std::string
caption (
void)
const;
70 inline const std::string &
rawCaption (
void)
const {
return mRawCaption;}
77 inline uint16_t
anchor (
void)
const {
return mAnchorPos;}
87 bool getCharWithAttrs (
const size_t inPos, std::string & outChar,
bool & outIsBold,
bool & outIsItalic, std::string & outColor)
const;
92 const SRTDuration inDuration,
const std::string & inRawCaption = std::string(),
93 const size_t inStartLineNum = 0,
const size_t inEndLineNum = 0)
94 { mSequenceNum = inSeqNum; mStartTime = inStartTime;
95 mDuration = inDuration; mRawCaption = inRawCaption;
96 mStartLineNum = inStartLineNum; mEndLineNum = inEndLineNum;
97 mAnchorPos = 0; mWindow.
clear(); mFirstCaption =
false;
104 {mStartLineNum = inStartLineNum; mEndLineNum = inEndLineNum;
return *
this;}
106 std::ostream &
printInfo (std::ostream & oss)
const;
107 std::ostream &
printSRT (std::ostream & oss)
const;
111 std::string mRawCaption;
117 size_t mStartLineNum;
131 static bool getWindow (
const std::string & inStr,
SRTWindow & outWndo, std::ostream & oss);
134 static std::string
anchorStr (
const uint16_t inAnchor,
const bool inIncludeRawValue =
false);
135 static std::string
anchorTag (
const uint16_t inAnchor);
153 bool reloadFrom (
const std::string & inSRTData);
169 inline size_t totalLines (
void)
const {
return mLines.size();}
170 inline std::string
lineAtOffset (
const size_t inOffset)
const {
return inOffset < mLines.size() ? mLines.at(inOffset) :
"";}
181 bool getWarning (
const size_t inIndex0,
size_t & outLineOffset, std::string & outMsg)
const;
182 bool getError (
size_t & outLineOffset, std::string & outMsg)
const;
183 std::ostream &
printErrors (std::ostream & oss,
const bool inIncludeWarnings =
true)
const;
184 std::ostream &
printSRT (std::ostream & oss)
const;
185 std::ostream &
printInfo (std::ostream & oss)
const;
208 #endif // __NTV2_SRT_H_
std::string asString(void) const
uint32_t captionCount(void) const
bool reloadFrom(const StringList &inSRTData)
std::string caption(void) const
SRTWindow & set(uint32_t inX1, uint32_t inY1, uint32_t inX2, uint32_t inY2)
SRTCaptionInfo & setLineNumbers(const size_t inStartLineNum, const size_t inEndLineNum)
std::ostream & printSRT(std::ostream &oss) const
size_t endLineNumber(void) const
SRTWindow & setTop(uint32_t inY1)
size_t linesParsed(void) const
std::map< size_t, std::string > ErrorMap
std::map< SRTTimestamp, SRTWindow > WindowMap
static bool getWindow(const std::string &inStr, SRTWindow &outWndo, std::ostream &oss)
bool durationAtStartTime(const SRTTimestamp inStartTime, SRTDuration &outDuration) const
std::string lineAtOffset(const size_t inOffset) const
SRTTimestamp maxStartTime(void) const
size_t endLineOffset(void) const
bool getWarning(const size_t inIndex0, size_t &outLineOffset, std::string &outMsg) const
size_t startLineOffset(void) const
SRTCaptionInfo & set(const SRTSeqNum inSeqNum, const SRTTimestamp inStartTime, const SRTDuration inDuration, const std::string &inRawCaption=std::string(), const size_t inStartLineNum=0, const size_t inEndLineNum=0)
uint32_t width(void) const
bool hasCaptionAtStartTime(const SRTTimestamp inStartTime) const
bool appendCaption(const SRTCaptionInfo &inCaptionInfo)
size_t totalLines(void) const
SRTWindow(uint32_t inX1=0, uint32_t inY1=0, uint32_t inX2=0, uint32_t inY2=0)
bool hasWindow(void) const
SRTWindow & setBottom(uint32_t inY2)
uint32_t right(void) const
SRTTimestamp startTime(void) const
bool getCharWithAttrs(const size_t inPos, std::string &outChar, bool &outIsBold, bool &outIsItalic, std::string &outColor) const
const ErrorMap & warnings(void) const
std::vector< std::string > StringList
static std::string durationStr(const SRTDuration inDurationMS, const bool inIncludeRawValue=false)
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
const ErrorMap & errors(void) const
SRTDuration duration(void) const
SRTTimestamp endTime(void) const
static std::string anchorTag(const uint16_t inAnchor)
SRTSeqNum minSeqNum(void) const
SRTWindow & setRight(uint32_t inX2)
bool getError(size_t &outLineOffset, std::string &outMsg) const
static std::string timestampStr(const SRTTimestamp inTS, const bool inIncludeRawValue=false)
std::ostream & print(std::ostream &oss) const
uint16_t anchor(void) const
SRTSeqNum maxSeqNum(void) const
static std::string anchorStr(const uint16_t inAnchor, const bool inIncludeRawValue=false)
uint32_t bottom(void) const
bool operator!=(const SRTWindow &inRHS) const
SRTTimestamp startTimeAtSeqNum(const SRTSeqNum inSeqNum) const
SRTCaptionInfo & reset(void)
SRTWindow & setLeft(uint32_t inX1)
bool hasWarnings(void) const
bool hasAnchor(void) const
std::ostream & printInfo(std::ostream &oss) const
SRTWindow & setTopLeft(uint32_t inX1, uint32_t inY1)
bool hasLineNumbers(void) const
const SRTWindow & window(void) const
std::map< SRTTimestamp, SRTDuration > DurationMap
SRTCaptionInfo & setWindow(const SRTWindow &inWindow)
std::ostream & operator<<(std::ostream &inOutStrm, const SRTWindow &inWindow)
std::ostream & printErrors(std::ostream &oss, const bool inIncludeWarnings=true) const
bool isFirstCaption(void) const
SRTTimestamp minStartTime(void) const
std::map< SRTTimestamp, uint32_t > AnchorMap
bool captionAtStartTime(const SRTTimestamp inStartTime, SRTCaptionInfo &outInfo) const
SRTCaptionInfo(const SRTSeqNum inSeqNum=0, const SRTTimestamp inStartTime=0, const SRTDuration inDuration=0, const std::string &inRawCaption=std::string(), const size_t inStartLineNum=0, const size_t inEndLineNum=0)
uint32_t height(void) const
bool hasErrors(void) const
bool operator==(const SRTWindow &inRHS) const
A collection of timed captions.
uint32_t left(void) const
size_t warningCount(void) const
static SRTDuration defaultMinimumDuration(void)
SRTSeqNum sequenceNum(void) const
std::map< SRTSeqNum, SRTTimestamp > TimestampMap
std::map< SRTTimestamp, SRTSeqNum > SeqNumMap
SRTWindow & setBottomRight(uint32_t inX2, uint32_t inY2)
SRTCaptionInfo & setAnchorPos(const uint16_t inAnchorPos)
std::ostream & printSRT(std::ostream &oss) const
const std::string & rawCaption(void) const
bool nextCaption(SRTTimestamp &inOutStartTime, SRTCaptionInfo &outInfo) const
size_t startLineNumber(void) const
std::map< SRTTimestamp, std::string > CaptionMap
std::ostream & printInfo(std::ostream &oss) const
static bool getTimestamp(const std::string &inStr, SRTTimestamp &outTS, std::ostream &oss=std::cerr)
static void setDefaultMinimumDuration(const SRTDuration inDuration)
bool hasCaptionAtSeqNum(const SRTSeqNum inSeqNum) const
SRTDuration totalDuration(void) const
SRTCaptionInfo & setFirstCaption(const bool inIsFirst=true)
bool hasAnchorTag(void) const
Detailed caption information.
size_t errorCount(void) const
std::map< SRTTimestamp, size_t > SourceMap