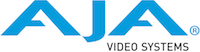 |
AJA NTV2 SDK
17.5.0.1658
NTV2 SDK 17.5.0.1658
|
Go to the documentation of this file.
9 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
14 #if defined(AJA_WINDOWS)
16 #elif defined(AJA_LINUX)
18 #elif defined(AJA_MAC)
20 #elif defined(AJA_BAREMETAL)
28 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
29 mpMutex =
new recursive_timed_mutex;
40 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
41 mpMutex =
new recursive_timed_mutex;
58 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
72 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
75 bool success = mpMutex->try_lock_for(std::chrono::milliseconds(timeout));
84 return mpImpl->Lock(timeout);
92 #if defined(AJA_USE_CPLUSPLUS11) && !defined(AJA_BAREMETAL)
96 return mpImpl->Unlock();
Declares the AJALockImpl class.
#define LOCK_TIME_INFINITE
Declares the AJALockImpl class.
Declares the AJALock class.
AJALock(const char *pName=NULL)
virtual AJAStatus Lock(uint32_t timeout=0xffffffff)
Declares the AJALockImpl class.
virtual AJALock & operator=(const AJALock &inLock)
AJAAutoLock(AJALock *pLock=NULL)
virtual AJAStatus Unlock()