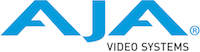 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
8 #ifndef NTV2REGEXPERT_H
9 #define NTV2REGEXPERT_H
19 #if defined (AJALinux)
25 #define YesNo(__x__) ((__x__) ? "Y" : "N")
26 #define OnOff(__x__) ((__x__) ? "On" : "Off")
27 #define SetNotset(__x__) ((__x__) ? "Set" : "Not Set")
28 #define EnabDisab(__x__) ((__x__) ? "Enabled" : "Disabled")
29 #define OddEven(__x__) ((__x__) ? "Odd" : "Even")
30 #define DisabEnab(__x__) ((__x__) ? "Disabled" : "Enabled")
31 #define ActInact(__x__) ((__x__) ? "Active" : "Inactive")
32 #define SuppNotsupp(__x__) ((__x__) ? "Supported" : "Unsupported")
33 #define PresNotPres(__x__) ((__x__) ? "Present" : "Not Present")
34 #define ThruDeviceOrBypassed(__x__) ((__x__) ? "Thru Device" : "Device Bypassed")
38 #define kRegClass_NULL std::string ()
39 #define kRegClass_AES std::string ("kRegClass_AES")
40 #define kRegClass_Analog std::string ("kRegClass_Analog")
41 #define kRegClass_Anc std::string ("kRegClass_Anc")
42 #define kRegClass_Audio std::string ("kRegClass_Audio")
43 #define kRegClass_Aux std::string ("kRegClass_Aux")
44 #define kRegClass_Channel1 std::string ("kRegClass_Channel1")
45 #define kRegClass_Channel2 std::string ("kRegClass_Channel2")
46 #define kRegClass_Channel3 std::string ("kRegClass_Channel3")
47 #define kRegClass_Channel4 std::string ("kRegClass_Channel4")
48 #define kRegClass_Channel5 std::string ("kRegClass_Channel5")
49 #define kRegClass_Channel6 std::string ("kRegClass_Channel6")
50 #define kRegClass_Channel7 std::string ("kRegClass_Channel7")
51 #define kRegClass_Channel8 std::string ("kRegClass_Channel8")
52 #define kRegClass_CSC std::string ("kRegClass_CSC")
53 #define kRegClass_DMA std::string ("kRegClass_DMA")
54 #define kRegClass_HDMI std::string ("kRegClass_HDMI")
55 #define kRegClass_HDR std::string ("kRegClass_HDR")
56 #define kRegClass_Input std::string ("kRegClass_Input")
57 #define kRegClass_Info std::string ("kRegClass_Info")
58 #define kRegClass_Interrupt std::string ("kRegClass_Interrupt")
59 #define kRegClass_IP std::string ("kRegClass_IP")
60 #define kRegClass_LUT std::string ("kRegClass_LUT")
61 #define kRegClass_Mixer std::string ("kRegClass_Mixer")
62 #define kRegClass_Output std::string ("kRegClass_Output")
63 #define kRegClass_ReadOnly std::string ("kRegClass_ReadOnly")
64 #define kRegClass_Routing std::string ("kRegClass_Routing")
65 #define kRegClass_SDIError std::string ("kRegClass_SDIError")
66 #define kRegClass_Serial std::string ("kRegClass_Serial")
67 #define kRegClass_Timecode std::string ("kRegClass_Timecode")
68 #define kRegClass_Timing std::string ("kRegClass_Timing")
69 #define kRegClass_Video std::string ("kRegClass_Video")
70 #define kRegClass_Virtual std::string ("kRegClass_Virtual")
71 #define kRegClass_VPID std::string ("kRegClass_VPID")
72 #define kRegClass_WriteOnly std::string ("kRegClass_WriteOnly")
73 #define kRegClass_XptROM std::string ("kRegClass_XptROM")
74 #define kRegClass_NTV4FrameStore std::string ("kRegClass_NTV4FrameStore")
77 #define kIncludeOtherRegs_None 0
78 #define kIncludeOtherRegs_VRegs 1 // Also include virtual regs
79 #define kIncludeOtherRegs_XptROM 2 // Also include crosspoint ROM regs
94 static std::string GetDisplayName (
const uint32_t inRegNum);
110 static bool IsRegisterInClass (
const uint32_t inRegNum,
const std::string & inClassName);
132 static NTV2StringSet GetRegisterClasses (
const uint32_t inRegNum,
const bool inRemovePrefix =
false);
138 static NTV2RegNumSet GetRegistersForClass (
const std::string & inClassName);
165 static NTV2RegNumSet GetRegistersWithName (
const std::string & inName,
const int inSearchStyle = EXACTMATCH);
176 static NTV2InputCrosspointID GetInputCrosspointID (
const uint32_t inXptRegNum,
const uint32_t inMaskIndex);
185 static bool GetCrosspointSelectGroupRegisterInfo (
const NTV2InputCrosspointID inInputXpt, uint32_t & outXptRegNum, uint32_t & outMaskIndex);
187 static const int CONTAINS = 0;
188 static const int STARTSWITH = 1;
189 static const int ENDSWITH = 2;
190 static const int EXACTMATCH = 3;
191 static bool IsAllocated(
void);
199 static bool Allocate(
void);
207 static bool Deallocate(
void);
211 #endif // NTV2REGEXPERT_H
static bool IsReadOnly(const uint32_t inRegNum)
Defines the import/export macros for producing DLLs or LIBs.
#define kIncludeOtherRegs_None
std::set< std::string > NTV2StringSet
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
#define kRegClass_WriteOnly
static bool IsWriteOnly(const uint32_t inRegNum)
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
NTV2RegisterNumberSet NTV2RegNumSet
A set of distinct NTV2RegisterNumbers.
Enumerations for controlling NTV2 devices.
Declares numerous NTV2 utility functions.
NTV2InputCrosspointID
Identifies a widget input that potentially can accept a signal emitted from another widget's output (...
#define kRegClass_ReadOnly
I provide "one-stop shopping" for information about registers and their values.
Declares enums and structs used by all platform drivers and the SDK.
@ DEVICE_ID_NOTFOUND
Invalid or "not found".