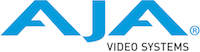 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
12 #if !defined(NTV2_BUILDING_DRIVER)
36 bool isProgressivePicture =
false;
37 bool isProgressiveTransport =
false;
38 bool isDualLink =
false;
39 bool isLevelA =
false;
40 bool isLevelB =
false;
44 bool isStereo =
false;
47 bool enableBT2020 =
false;
48 bool isMultiLink =
false;
61 if (! pOutVPID || ! pInVPIDSpec)
68 is3G = isLevelA || isLevelB;
96 isProgressiveTransport = isProgressivePicture;
99 (!isRGB && isDualLink && !isTSI) ||
102 isProgressiveTransport =
false;
109 isProgressiveTransport =
false;
114 isProgressiveTransport =
false;
119 isProgressiveTransport =
false;
126 switch (outputFormat)
422 byte2 |= (transferCharacteristics << 4);
425 byte2 |= isProgressivePicture ? (1UL << 6) : 0;
428 byte2 |= isProgressiveTransport ? (1UL << 7) : 0;
450 if (is6G || isLevelA)
459 if(is6G || is12G || isLevelA)
471 if (isLevelB && isDualLink)
478 highBit = (colorimetry&0x2)>>1;
479 lowBit = colorimetry&0x1;
483 if (is6G || isLevelA)
484 byte3 |= (highBit << 5);
486 byte3 |= (highBit << 7);
488 byte3 |= (lowBit << 4);
493 if(is6G || is12G || isLevelA)
495 byte3 |= (highBit << 5);
496 byte3 |= (lowBit << 4);
500 byte3 |= (highBit << 7);
501 byte3 |= (lowBit << 4);
576 byte4 |= vpidChannel << 5;
578 byte4 |= vpidChannel << 6;
583 byte4 |= vpidChannel << 6;
598 byte4 |= (luminance << 4);
621 *pOutVPID = ((
ULWord)byte1 << 24) | ((
ULWord)byte2 << 16) | ((
ULWord)byte3 << 8) | byte4;
@ NTV2_FORMAT_3840x2160p_6000
@ NTV2_FORMAT_4096x2160psf_2500
@ NTV2_FORMAT_4x1920x1080p_6000
@ VPIDStandard_1080_DualLink_3Gb
@ NTV2_FORMAT_3840x2160psf_2500
@ NTV2_FORMAT_1080psf_2398
@ NTV2_FORMAT_4096x2160p_2400
@ NTV2_FBF_ARGB
See 8-Bit ARGB, RGBA, ABGR Formats.
@ NTV2_FORMAT_4096x2160p_12000
NTV2VPIDTransferCharacteristics transferCharacteristics
Describes the transfer characteristics.
@ NTV2_FORMAT_4x4096x2160p_4800
@ NTV2_FORMAT_4x4096x2160p_2398
@ NTV2_FBF_12BIT_RGB_PACKED
See 12-Bit Packed RGB.
bool SetVPIDFromSpec(ULWord *const pOutVPID, const VPIDSpec *const pInVPIDSpec)
Generates a VPID based on the supplied specification.
@ NTV2_FORMAT_4096x2160p_11988
bool isMultiLink
If true, the video stream is 12G -> 3G multi-link.
NTV2VideoFormat videoFormat
Specifies the format of the video stream.
@ NTV2_FORMAT_4096x2160p_2500
@ NTV2_FRAMERATE_6000
60 frames per second
#define NTV2_VIDEO_FORMAT_HAS_PROGRESSIVE_PICTURE(__f__)
@ NTV2_FORMAT_525psf_2997
@ NTV2_FORMAT_4x2048x1080p_4795
@ NTV2_FBF_10BIT_DPX_LE
10-Bit DPX Little-Endian
@ NTV2_FORMAT_4096x2160psf_2398
@ NTV2_FRAMERATE_2997
Fractional rate of 30,000 frames per 1,001 seconds.
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
@ NTV2_FORMAT_1080p_2K_6000_B
@ NTV2_FBF_RGBA
See 8-Bit ARGB, RGBA, ABGR Formats.
@ NTV2_FORMAT_1080psf_2K_2398
@ VPIDStandard_1080_Stereo_3Gb
@ NTV2_FORMAT_1080p_2K_4800_A
@ NTV2_FBF_48BIT_RGB
See 48-Bit RGB.
bool isOutputLevelA
If true, the video stream will leave the device as a level A signal.
@ NTV2_FORMAT_1080p_2K_3000
@ NTV2_FORMAT_4096x2160psf_2997
@ NTV2_FORMAT_4x2048x1080p_2997
@ NTV2_FBF_10BIT_ARGB
10-Bit ARGB
@ NTV2_FORMAT_4x4096x2160p_2500
bool isTwoSampleInterleave
If true, the video stream is in SMPTE 425-3 two sample interleave format.
@ NTV2_FORMAT_4x4096x2160p_4795
@ NTV2_FORMAT_4x1920x1080p_2997
@ NTV2_FORMAT_4x1920x1080p_2500
@ NTV2_FORMAT_4x2048x1080p_4800
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
@ NTV2_FORMAT_4x3840x2160p_2500
#define NTV2_IS_2K_1080_VIDEO_FORMAT(__f__)
@ NTV2_FBF_10BIT_DPX
See 10-Bit RGB - DPX Format.
@ NTV2_FORMAT_4x3840x2160p_5000_B
@ VPIDStandard_483_576_3Gb
NTV2VPIDLuminance luminance
Describes the luminance and color difference.
bool isStereo
If true, the video stream is part of a stereo pair.
@ NTV2_FORMAT_4x3840x2160p_2398
@ NTV2_FBF_24BIT_RGB
See 24-Bit RGB.
@ NTV2_FORMAT_4x4096x2160p_6000_B
@ NTV2_FRAMERATE_2500
25 frames per second
@ NTV2_FORMAT_1080p_2K_4795_A
@ NTV2_FORMAT_3840x2160p_2500
NTV2FrameRate
Identifies a particular video frame rate.
@ VPIDStandard_2160_DualLink
@ VPIDStandard_1080_Dual_3Gb
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
Contains all the information needed to generate a valid VPID.
@ NTV2_FORMAT_4x4096x2160p_2997
@ NTV2_FRAMERATE_4800
48 frames per second
#define NTV2_IS_4K_VIDEO_FORMAT(__f__)
#define NTV2_IS_UHD2_FULL_VIDEO_FORMAT(__f__)
@ NTV2_FORMAT_4096x2160psf_2400
Declares functions for the C implementations of VPID generation from a VPIDSpec.
VPIDChannel vpidChannel
Specifies the channel number of the video stream.
@ NTV2_FRAMERATE_2400
24 frames per second
@ NTV2_FORMAT_4x2048x1080psf_2398
@ NTV2_FBF_10BIT_RGB_PACKED
10-Bit Packed RGB
@ NTV2_FORMAT_4x2048x1080p_2398
@ NTV2_FORMAT_1080psf_2K_2500
@ NTV2_FORMAT_4x2048x1080psf_2400
@ NTV2_FORMAT_1080p_5994_B
@ NTV2_FORMAT_1080psf_3000_2
@ NTV2_FORMAT_1080p_2K_2400
@ VPIDStandard_4320_DualLink_12Gb
@ NTV2_FORMAT_4x2048x1080p_3000
bool isOutputLevelB
If true, the video stream will leave the device as a level B signal.
@ NTV2_FORMAT_4096x2160p_4795
@ NTV2_FRAMERATE_2398
Fractional rate of 24,000 frames per 1,001 seconds.
@ NTV2_FORMAT_4x4096x2160p_3000
#define NTV2_IS_WIRE_FORMAT(__f__)
@ NTV2_FORMAT_4x3840x2160p_5994
@ NTV2_FORMAT_4096x2160p_2398
@ VPIDStandard_2160_QuadLink_3Ga
@ NTV2_FORMAT_3840x2160psf_3000
@ NTV2_FORMAT_4x2048x1080p_5000
@ NTV2_FBF_24BIT_BGR
See 24-Bit BGR.
@ NTV2_FORMAT_4096x2160p_6000
@ VPIDStandard_720_1080_Stereo
#define NTV2_IS_4K_HFR_VIDEO_FORMAT(__f__)
@ NTV2_FORMAT_4x1920x1080p_3000
@ NTV2_FORMAT_4x3840x2160p_2997
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
#define NTV2_IS_SQUARE_DIVISION_FORMAT(__f__)
Declares numerous NTV2 utility functions.
@ NTV2_FORMAT_1080p_5000_B
#define NTV2_IS_VALID_FBF(__s__)
@ VPIDStandard_4320_QuadLink_12Gb
@ NTV2_FORMAT_4x3840x2160p_5000
@ NTV2_FORMAT_1080p_2K_5994_A
@ VPIDStandard_2160_Single_12Gb
@ VPIDStandard_1080_Stereo_3Ga
#define NTV2_IS_HD_VIDEO_FORMAT(__f__)
@ NTV2_FORMAT_625psf_2500
@ VPIDStandard_2160_DualLink_12Gb
@ NTV2_FORMAT_1080p_2K_2500
@ NTV2_FORMAT_4x4096x2160p_4800_B
@ VPIDStandard_1080_Single_6Gb
@ VPIDStandard_1080_Dual_3Ga
@ NTV2_FORMAT_1080p_6000_A
@ NTV2_FORMAT_3840x2160psf_2398
@ NTV2_FORMAT_1080p_2K_2398
@ NTV2_FORMAT_3840x2160p_2997
@ NTV2_FORMAT_4096x2160p_4800
@ VPIDStandard_2160_Single_6Gb
@ NTV2_FORMAT_3840x2160psf_2400
@ VPIDStandard_2160_QuadDualLink_3Gb
@ NTV2_FORMAT_1080psf_2K_2400
#define NTV2_IS_PSF_VIDEO_FORMAT(__f__)
@ NTV2_FORMAT_3840x2160p_5994
@ NTV2_FORMAT_4x4096x2160p_2400
@ NTV2_FBF_8BIT_YCBCR_YUY2
See Alternate 8-Bit YCbCr ('YUY2').
@ NTV2_FORMAT_4x4096x2160p_6000
@ NTV2_FORMAT_3840x2160p_2398
#define NTV2_IS_QUAD_QUAD_FORMAT(__f__)
@ NTV2_FORMAT_4x1920x1080psf_2398
@ NTV2_FORMAT_1080p_2K_6000_A
@ NTV2_FBF_10BIT_YCBCR
See 10-Bit YCbCr Format.
bool isDualLink
If true, the video stream is part of a SMPTE 372 dual link signal.
bool enableBT2020
If true, the VPID will insert BT.2020 data.
bool isRightEye
If true, the video stream is the right eye of a stereo pair.
@ NTV2_FORMAT_4096x2160p_3000
bool isOutput12G
If true, the transport on the wire is 12G.
@ NTV2_FORMAT_4x1920x1080psf_2500
@ NTV2_FRAMERATE_5994
Fractional rate of 60,000 frames per 1,001 seconds.
@ NTV2_FORMAT_4x3840x2160p_2400
@ NTV2_FORMAT_4x4096x2160p_5000_B
@ NTV2_FORMAT_4x2048x1080p_2500
@ NTV2_FORMAT_4x1920x1080p_5994
@ NTV2_FORMAT_4x4096x2160p_5000
@ NTV2_FORMAT_4x3840x2160p_6000
NTV2VPIDRGBRange rgbRange
Describes the RGB range as full or SMPTE.
@ VPIDStandard_720_Stereo_3Ga
@ NTV2_FBF_10BIT_YCBCR_DPX
See 10-Bit YCbCr - DPX Format.
@ NTV2_FRAMERATE_UNKNOWN
Represents an unknown or invalid frame rate.
NTV2FrameBufferFormat pixelFormat
Specifies the pixel format of the source of the video stream.
@ NTV2_FORMAT_1080p_5000_A
@ NTV2_FORMAT_4x2048x1080p_6000
@ NTV2_FORMAT_3840x2160psf_2997
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
@ NTV2_FORMAT_4096x2160p_5000
NTV2VPIDColorimetry colorimetry
Describes the Colorimetry.
bool isRGBOnWire
If true, the transport on the wire is RGB.
@ NTV2_FORMAT_4x1920x1080p_5000
@ NTV2_FORMAT_4x1920x1080p_2398
NTV2VPIDTransferCharacteristics
@ NTV2_FORMAT_1080p_6000_B
@ NTV2_FORMAT_4x4096x2160p_5994_B
@ VPIDStandard_1080_DualLink
@ VPIDStandard_720_Stereo_3Gb
@ NTV2_FORMAT_4096x2160p_2997
@ NTV2_FORMAT_4x3840x2160p_5994_B
#define NTV2_IS_QUAD_FRAME_FORMAT(__f__)
@ NTV2_FORMAT_1080p_5994_A
@ NTV2_FORMAT_4x3840x2160p_6000_B
@ NTV2_FORMAT_4x2048x1080p_5994
bool isOutput6G
If true, the transport on the wire is 6G.
@ NTV2_FORMAT_4x3840x2160p_3000
@ NTV2_FORMAT_1080p_2K_5994_B
#define NTV2_IS_4K_4096_VIDEO_FORMAT(__f__)
@ NTV2_FORMAT_3840x2160p_5000
@ NTV2_FORMAT_4096x2160psf_3000
@ NTV2_FORMAT_1080p_2K_5000_A
@ NTV2_FRAMERATE_5000
50 frames per second
#define NTV2_IS_720P_VIDEO_FORMAT(__f__)
@ NTV2_FORMAT_4x4096x2160p_4795_B
@ NTV2_FORMAT_4x2048x1080psf_2500
@ NTV2_FBF_10BIT_RGB
See 10-Bit RGB Format.
#define NTV2_IS_UHD2_VIDEO_FORMAT(__f__)
@ NTV2_FRAMERATE_4795
Fractional rate of 48,000 frames per 1,001 seconds.
@ NTV2_FRAMERATE_3000
30 frames per second
@ NTV2_FORMAT_1080p_2K_5000_B
@ NTV2_FORMAT_1080psf_2997_2
@ NTV2_FORMAT_4x4096x2160p_5994
@ NTV2_FORMAT_4x2048x1080p_2400
@ NTV2_FORMAT_4x1920x1080p_2400
@ NTV2_FORMAT_4x1920x1080psf_2400
@ NTV2_FORMAT_1080psf_2500_2
@ NTV2_VPID_Luminance_YCbCr
VPIDAudio audioCarriage
Specifies how audio is carried in additional channels.
@ NTV2_FORMAT_3840x2160p_2400
@ NTV2_FBF_ABGR
See 8-Bit ARGB, RGBA, ABGR Formats.
@ NTV2_FORMAT_3840x2160p_3000
@ NTV2_FORMAT_1080psf_2400
@ NTV2_FORMAT_4096x2160p_5994
@ NTV2_FORMAT_1080p_2K_2997
bool useChannel
If true, the following vpidChannel value should be inserted into th VPID.