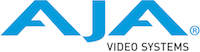 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
8 #ifndef NTV2MACDRIVERINTERFACE_H
9 #define NTV2MACDRIVERINTERFACE_H
52 const ULWord inFrameNumber,
54 const ULWord inCardOffsetBytes,
56 const bool inSynchronous =
true);
60 const ULWord inFrameNumber,
62 const ULWord inCardOffsetBytes,
64 const ULWord inNumSegments,
65 const ULWord inSegmentHostPitch,
66 const ULWord inSegmentCardPitch,
67 const bool inSynchronous =
true);
71 const bool inIsTarget,
72 const ULWord inFrameNumber,
73 const ULWord inCardOffsetBytes,
75 const ULWord inNumSegments,
76 const ULWord inSegmentHostPitch,
77 const ULWord inSegmentCardPitch,
86 #if !defined(NTV2_DEPRECATE_16_0)
98 #endif // !defined(NTV2_DEPRECATE_16_0)
108 #if !defined(NTV2_NULL_DEVICE)
112 #endif // !defined(NTV2_NULL_DEVICE)
116 AJA_VIRTUAL inline io_connect_t GetIOConnect (
void)
const {
return mConnection;}
117 io_connect_t mConnection;
128 #endif // NTV2MACDRIVERINTERFACE_H
virtual bool MapRegisters(void)
enum _INTERRUPT_ENUMS_ INTERRUPT_ENUMS
virtual bool GetAudioOutputMode(NTV2_GlobalAudioPlaybackMode *mode)
virtual bool KernelLog(void *dataPtr, UInt32 dataSize)
NTV2_DriverDebugMessageSet
virtual ~CNTV2MacDriverInterface()
My destructor.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
virtual bool AutoCirculate(AUTOCIRCULATE_DATA &autoCircData)
Sends an AutoCirculate command to the NTV2 driver.
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
virtual bool OpenLocalPhysical(const UWord inDeviceIndex)
Opens the local/physical device connection.
virtual bool WaitForInterrupt(const INTERRUPT_ENUMS type, const ULWord timeout=50)
virtual bool GetInterruptCount(const INTERRUPT_ENUMS eInterrupt, ULWord &outCount)
Answers with the number of interrupts of the given type processed by the driver.
NTV2_GlobalAudioPlaybackMode
virtual bool SystemStatus(void *dataPtr, SystemStatusCode systemStatusCode)
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
virtual bool MapXena2Flash(void)
virtual std::string GetConnectionType(void) const
All new NTV2 structs start with this common header.
virtual bool RestoreHardwareProcampRegisters(void)
Declares MacOS-only enums used by the Mac driver and the SDK.
virtual bool WaitForChangeEvent(UInt32 timeout=0)
virtual bool ControlDriverDebugMessages(NTV2_DriverDebugMessageSet, bool)
virtual bool ConfigureInterrupt(bool, INTERRUPT_ENUMS)
virtual bool MapMemory(const MemoryType memType, void **memPtr)
virtual bool SystemControl(void *dataPtr, SystemControlCode systemControlCode)
virtual bool UnmapXena2Flash(void)
#define NTV2_SHOULD_BE_DEPRECATED(__f__)
#define NTV2_DEPRECATED_f(__f__)
virtual bool MapFrameBuffers(void)
virtual bool SetStreamingApplication(const ULWord appType, const int32_t pid)
Sets the four-CC type and process ID of the application that should "own" the AJA device (i....
virtual bool NTV2Message(NTV2_HEADER *pInMessage)
Sends a message to the NTV2 driver (the new, improved, preferred way).
virtual bool AcquireStreamForApplicationWithReference(ULWord inApplicationType, int32_t inProcessID)
A reference-counted version of CNTV2DriverInterface::AcquireStreamForApplication useful for process g...
virtual bool UnmapRegisters(void)
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
A Mac-specific implementation of CNTV2DriverInterface.
CNTV2MacDriverInterface()
My default constructor.
virtual bool DmaTransfer(const NTV2DMAEngine inDMAEngine, const bool inIsRead, const ULWord inFrameNumber, ULWord *pFrameBuffer, const ULWord inCardOffsetBytes, const ULWord inByteCount, const bool inSynchronous=(!(0)))
Transfers data between the AJA device and the host. This function will block and not return to the ca...
virtual bool UnmapFrameBuffers(void)
virtual bool SetAudioOutputMode(NTV2_GlobalAudioPlaybackMode mode)
Declares enums and structs used by all platform drivers and the SDK.
virtual bool ReleaseStreamForApplicationWithReference(ULWord inApplicationType, int32_t inProcessID)
A reference-counted version of CNTV2DriverInterface::ReleaseStreamForApplication useful for process g...
I'm the base class that undergirds the platform-specific derived classes (from which CNTV2Card is ult...
Declares the CNTV2DriverInterface base class.
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
virtual ULWord GetPCISlotNumber(void) const
virtual bool CloseLocalPhysical(void)
Releases host resources associated with the local/physical device connection.