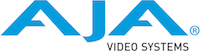 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
16 : mPrecision (inPrecision)
53 mStartTime = mStopTime = 0;
69 return uint32_t(mStopTime - mStartTime);
73 #define __fDEC(__x__,__w__,__p__) std::dec << std::fixed << std::setw(__w__) << std::setprecision(__p__) << (__x__)
77 const double secs(
ETSecs());
80 const double mins (secs / 60.0);
83 const double hrs (mins / 60.0);
86 const double days (hrs / 24.0);
87 oss <<
__fDEC(days,4,1) <<
" days";
90 oss <<
__fDEC(hrs,4,1) <<
" hrs";
93 oss <<
__fDEC(mins,4,1) <<
" mins";
96 oss <<
__fDEC(secs,4,1) <<
" secs";
97 else if (secs >= 1.0E-03)
98 oss <<
__fDEC(secs*1.0E+3,4,1) <<
" msec";
99 else if (secs >= 1.0E-06)
100 oss <<
__fDEC(secs*1.0E+6,4,1) <<
" usec";
101 else if (secs >= 1.0E-09)
102 oss <<
__fDEC(secs*1.0E+9,4,1) <<
" nsec";
103 else if (secs >= 1.0E-12)
104 oss <<
__fDEC(secs*1.0E+12,4,1) <<
" psec";
bool IsRunning(void) const
AJATimer(const AJATimerPrecision precision=AJATimerPrecisionMilliseconds)
Declares the AJATime class.
static uint64_t GetSystemMicroseconds(void)
Returns the current value of the host's high-resolution clock, in microseconds.
std::ostream & Print(std::ostream &oss) const
Declares the AJATimer class.
double ETSecs(void) const
static uint64_t GetSystemNanoseconds(void)
Returns the current value of the host's high-resolution clock, in nanoseconds.
System specific functions.
Private include file for all ajabase sources.
static std::string PrecisionName(const AJATimerPrecision precision, const bool longName=true)
#define __fDEC(__x__, __w__, __p__)
static double PrecisionSecs(const AJATimerPrecision precision)
@ AJATimerPrecisionMicroseconds
@ AJATimerPrecisionNanoseconds
uint32_t ElapsedTime(void) const
@ AJATimerPrecisionMilliseconds
static uint64_t GetSystemMilliseconds(void)
Returns the current value of the host's high-resolution clock, in milliseconds.