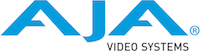 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
8 #ifndef AJA_THREAD_IMPL_H
9 #define AJA_THREAD_IMPL_H
39 AJAStatus Attach(AJAThreadFunction* pThreadFunction,
void* pUserContext);
64 #endif // AJA_THREAD_IMPL_H
AJAThreadPriority mPriority
static uint64_t GetThreadId()
AJAStatus SetThreadName(const char *name)
AJAThreadFunction * mThreadFunc
AJAStatus Kill(uint32_t exitCode)
Declares the AJALock class.
pthread_cond_t mStartCond
pthread_mutex_t mStartMutex
System specific functions.
Private include file for all ajabase sources.
pthread_mutex_t mExitMutex
static void * ThreadProcStatic(void *pThreadImplContext)
AJAThreadImpl(AJAThread *pThreadContext)
AJAStatus Stop(uint32_t timeout=0xffffffff)
AJAThread * mpThreadContext
AJAStatus SetPriority(AJAThreadPriority threadPriority)
AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
Declares the AJAThread class.
AJAStatus GetPriority(AJAThreadPriority *pThreadPriority)
AJAStatus SetRealTime(AJAThreadRealTimePolicy policy, int priority)