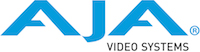 |
AJA NTV2 SDK
17.5.0.1242
NTV2 SDK 17.5.0.1242
|
Go to the documentation of this file.
17 const int maxbufsize = 256;
18 char buffer[maxbufsize];
19 std::string result =
"";
20 FILE* pipe = popen(cmd,
"r");
23 throw std::runtime_error(
"popen() failed!");
30 if (fgets(buffer, maxbufsize, pipe) !=
NULL)
53 std::string
aja_procfs(
const char* procfs_file,
const char* value_key)
65 std::ostringstream oss;
66 oss <<
"cat /proc/" << procfs_file <<
" | grep '" << value_key <<
"' | head -n 1 | cut -d ':' -f 2 | xargs | tr -d '\n' | tr -s ' '";
68 return aja_cmd(oss.str().c_str());
77 std::ostringstream oss;
78 oss <<
"date -d \"`cut -f1 -d. /proc/uptime` seconds ago\" \"+%Y-%m-%d %H:%M:%S\"";
79 out =
aja_cmd(oss.str().c_str());
84 out =
aja_cmd(
"uptime -s 2>/dev/null");
94 out =
aja_cmd(
"lsb_release -d -s 2>/dev/null");
104 out =
aja_cmd(
"cat /etc/redhat-release 2>/dev/null");
108 out =
aja_cmd(
"cat /etc/os-release 2>/dev/null | grep 'PRETTY_NAME' | head -n 1 | cut -d '=' -f 2 | tr -d '\"' | tr -d '\n'");
119 out =
aja_cmd(
"lsb_release -r -s 2>/dev/null");
127 out =
aja_cmd(
"cat /etc/os-release 2>/dev/null | grep 'VERSION_ID' | head -n 1 | cut -d '=' -f 2 | tr -d '\"' | tr -d '\n'");
136 std::vector<std::string>& outFoundDevices)
138 if (inDevicePart.size() >= 2)
143 if (inDevicePart.find(
"SVendor") != inDevicePart.end())
144 vend = inDevicePart.at(
"SVendor");
145 else if (inDevicePart.find(
"Vendor") != inDevicePart.end())
146 vend = inDevicePart.at(
"Vendor");
148 if (inDevicePart.find(
"SDevice") != inDevicePart.end())
149 device = inDevicePart.at(
"SDevice");
150 else if (inDevicePart.find(
"Device") != inDevicePart.end())
151 device = inDevicePart.at(
"Device");
153 outFoundDevices.push_back(vend +
" " + device);
160 out =
aja_cmd(
"lspci -vmm | grep VGA -A 4");
179 std::ostringstream oss;
180 std::vector<std::string> lines =
aja::split(out,
'\n');
181 if (lines.empty() ==
false)
183 std::vector<std::string>::iterator it;
184 std::vector<std::string> foundDevices;
185 std::map<std::string, std::string> deviceParts;
186 for(it=lines.begin();it!=lines.end();++it)
188 if (*it ==
"--" || *it ==
"")
190 if (deviceParts.size() >= 2)
198 std::vector<std::string> cols =
aja::split(*it,
':');
202 std::string val = cols.at(1);
204 for(
size_t i=2;i<cols.size();++i)
206 val = val +
":" + cols.at(i);
215 else if (key ==
"Vendor" || key ==
"Device" ||
216 key ==
"SVendor" || key ==
"SDevice")
218 deviceParts[key] = val;
223 if (deviceParts.size() >= 2)
229 for(
size_t i=0;i<foundDevices.size();++i)
235 oss << foundDevices.at(i);
256 static char tmp_buf[4096];
261 gethostname(tmp_buf,
sizeof(tmp_buf));
281 long int numProcs = sysconf(_SC_NPROCESSORS_ONLN);
282 std::ostringstream num_cores;
283 num_cores << numProcs;
291 std::string memTotalStr =
aja_procfs(
"meminfo",
"MemTotal");
292 int64_t memtotalbytes=0;
293 if (memTotalStr.find(
" kB") != std::string::npos)
297 std::istringstream(memTotalStr) >> memtotalbytes;
298 memtotalbytes *= 1024;
303 std::istringstream(memTotalStr) >> memtotalbytes;
306 std::string memFreeStr =
aja_procfs(
"meminfo",
"MemFree");
307 int64_t memfreebytes=0;
308 if (memFreeStr.find(
" kB") != std::string::npos)
312 std::istringstream(memFreeStr) >> memfreebytes;
313 memfreebytes *= 1024;
318 std::istringstream(memFreeStr) >> memfreebytes;
321 std::string unitsLabel;
322 double divisor = 1.0;
339 divisor = 1073741824.0;
343 int64_t memusedbytes = memtotalbytes - memfreebytes;
345 std::ostringstream t,u,f;
346 t << int64_t(memtotalbytes / divisor) <<
" " << unitsLabel;
347 u << int64_t(memusedbytes / divisor) <<
" " << unitsLabel;
348 f << int64_t(memfreebytes / divisor) <<
" " << unitsLabel;
366 const char* homePath = getenv(
"HOME");
367 if (homePath !=
NULL)
@ AJA_SystemInfoSection_Path
Declares the AJASystemInfo class.
@ AJA_SystemInfoMemoryUnit_Megabytes
@ AJA_SystemInfoSection_CPU
std::string & strip(std::string &str, const std::string &ws)
void split(const std::string &str, const char delim, std::vector< std::string > &elems)
@ AJA_SystemInfoTag_Path_Firmware
@ AJA_SystemInfoTag_OS_KernelVersion
std::string aja_procfs(const char *procfs_file, const char *value_key)
@ AJA_SystemInfoTag_Path_Applications
virtual ~AJASystemInfoImpl()
void get_vendor_and_device(std::map< std::string, std::string > &inDevicePart, std::vector< std::string > &outFoundDevices)
@ AJA_SystemInfoMemoryUnit_Kilobytes
@ AJA_SystemInfoTag_CPU_Type
std::string aja_productname()
std::string aja_cmd(const char *cmd)
@ AJA_SystemInfoTag_CPU_NumCores
std::string & replace(std::string &str, const std::string &from, const std::string &to)
@ AJA_SystemInfoTag_System_Name
std::string aja_osversion()
std::map< int, std::string > mValueMap
@ AJA_SystemInfoTag_System_BootTime
@ AJA_SystemInfoTag_OS_Version
@ AJA_SystemInfoTag_Mem_Used
@ AJA_SystemInfoSection_GPU
@ AJA_SystemInfoTag_Path_PersistenceStoreUser
AJASystemInfoImpl(int units)
@ AJA_SystemInfoTag_Path_PersistenceStoreSystem
Declares the AJAFileIO class.
@ AJA_SystemInfoMemoryUnit_Gigabytes
@ AJA_SystemInfoTag_OS_ProductName
static bool FileExists(const std::wstring &fileName)
@ AJA_SystemInfoSection_OS
@ AJA_SystemInfoTag_Path_Utilities
@ AJA_SystemInfoTag_Path_UserHome
virtual AJAStatus Rescan(AJASystemInfoSections sections)
@ AJA_SystemInfoSection_Mem
@ AJA_SystemInfoTag_Mem_Free
@ AJA_SystemInfoTag_GPU_Type
std::string aja_getgputype()
@ AJA_SystemInfoTag_OS_VersionBuild
@ AJA_SystemInfoMemoryUnit_Bytes
@ AJA_SystemInfoSection_System
@ AJA_SystemInfoTag_Mem_Total
Declares the AJASystemInfoImpl class.
@ AJA_SystemInfoTag_System_Model