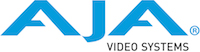 |
AJA NTV2 SDK
17.5.0.1242
NTV2 SDK 17.5.0.1242
|
Go to the documentation of this file.
70 if (timeout == 0xffffffff)
81 DWORD returnCode = WaitForSingleObject(
mhThreadHandle, (DWORD)timeout);
83 if (returnCode == WAIT_TIMEOUT)
88 else if (returnCode != WAIT_OBJECT_0)
137 if (returnCode == WAIT_OBJECT_0)
178 switch (threadPriority)
190 success = SetThreadPriority(
mhThreadHandle, THREAD_PRIORITY_TIME_CRITICAL)?
true :
false;
193 success = SetThreadPriority(
mhThreadHandle, THREAD_PRIORITY_ABOVE_NORMAL)?
true :
false;
209 if(pTheadPPriority ==
NULL)
244 if (pThreadImpl ==
NULL)
279 return uint64_t(GetCurrentThreadId());
@ AJA_ThreadPriority_High
AJAThreadPriority mPriority
@ AJA_DebugSeverity_Warning
static uint64_t GetThreadId()
AJAStatus SetThreadName(const char *name)
@ AJA_DebugSeverity_Error
AJAThreadFunction * mThreadFunc
@ AJA_ThreadPriority_TimeCritical
@ AJA_ThreadPriority_Unknown
AJAStatus Kill(uint32_t exitCode)
@ AJA_ThreadPriority_Normal
#define AJA_REPORT(_index_, _severity_, _format_,...)
Declares the AJAThreadImpl class.
virtual AJAStatus ThreadRun()
@ AJA_ThreadPriority_AboveNormal
static void * ThreadProcStatic(void *pThreadImplContext)
AJAThreadImpl(AJAThread *pThreadContext)
AJAStatus Stop(uint32_t timeout=0xffffffff)
void AJAThreadFunction(AJAThread *pThread, void *pContext)
#define AJA_ASSERT(_expression_)
AJAStatus SetPriority(AJAThreadPriority threadPriority)
AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
AJAStatus GetPriority(AJAThreadPriority *pThreadPriority)
Declares the AJADebug class.
AJAStatus SetRealTime(AJAThreadRealTimePolicy policy, int priority)